本系列文章配套代码获取有以下两种途径:
-
通过百度网盘获取:
链接:https://pan.baidu.com/s/1XuxKa9_G00NznvSK0cr5qw?pwd=mnsj
提取码:mnsj
-
前往GitHub获取:
https://github.com/returu/PyTorch
逐元素操作:
常见的逐元素操作如下表所示:
函数 |
描述 |
abs/sqrt/rsqrt/exp/fmod/log/pow… | 绝对值/平方根/平方根倒数/指数/求余/对数/求幂等 |
cos/sin/asin/cosh… |
三角函数 |
ceil/round/floor/trunc/frac |
近似值运算,上取整/四舍五入/下去整/只保留整数部分/只保留小数部分 |
clamp(input,min,max) |
截断函数 |
# 使用full()创建一个全部都是3的,shape是2*2的tensor
>>> t = torch.full([2,2],3)
>>> t
tensor([[3, 3],
[3, 3]])
# 幂运算
>>> t.pow(3)
tensor([[27, 27],
[27, 27]])
>>> t**3
tensor([[27, 27],
[27, 27]])
# 平方根运算
>>> t.sqrt()
tensor([[1.7321, 1.7321],
[1.7321, 1.7321]])
# e为底的指数函数运算
>>> torch.exp(t)
tensor([[20.0855, 20.0855],
[20.0855, 20.0855]])
# 对数运算,log是以自然对数为底数的,以2为底的用log2,以10为底的用log10
>>> torch.log(t)
tensor([[1.0986, 1.0986],
[1.0986, 1.0986]])
# floor()往下取整数,ceil()往上取整数,trunc()取数据的整数部分,frac()取数据的小数部分,round()为四舍五入
>>> t = torch.tensor(3.54)
>>> t.floor(),t.ceil(),t.trunc(),t.frac(),t.round()
(tensor(3.), tensor(4.), tensor(3.), tensor(0.5400), tensor(4.))
>>> t = torch.tensor([[6.8, 1.1, 9.4],
... [9.2, 3.6, 8.2]])
# clamp(7)将<7的数据变为7
>>> t.clamp(7)
tensor([[7.0000, 7.0000, 9.4000],
[9.2000, 7.0000, 8.2000]])
# 将数据限定在(2,7),将<2的数据变为2,将>7的数据变为7
>>> t.clamp(2,7)
tensor([[6.8000, 2.0000, 7.0000],
[7.0000, 3.6000, 7.0000]])
数学运算:
-
基本数学运算:
-
加法:使用+运算符或torch.add()函数。 -
减法:使用–运算符或torch.sub()函数。 -
乘法:元素乘法:使用*运算符或torch.mul()函数。标量乘法:将一个标量与Tensor相乘,使用*运算符。 -
除法:元素除法:使用/运算符或torch.div()函数。标量除法:将一个标量除以Tensor的每个元素,或使用/运算符将一个Tensor除以一个标量。
>>> a = torch.rand(3,4)
>>> b = torch.rand(4)
>>> a+b
tensor([[0.7190, 0.3777, 0.1473, 0.3848],
[0.4840, 0.9010, 0.1591, 0.7213],
[0.8136, 0.6705, 0.1932, 0.7443]])
>>> torch.sub(a,b)
tensor([[-0.1437, 0.0237, 0.0916, 0.0508],
[-0.3786, 0.5469, 0.1034, 0.3873],
[-0.0490, 0.3165, 0.1375, 0.4103]])
-
矩阵乘法:
矩阵乘法有以下三种方式:
-
torch.mm(只适合于dim=2的tensor,因此不推荐使用) -
torch.matmul(),推荐使用此方法; -
符号@。
>>> a = torch.rand(2,2)
>>> a
tensor([[0.3238, 0.8680],
[0.6457, 0.4380]])
>>> b = torch.ones(2,2)
>>> b
tensor([[1., 1.],
[1., 1.]])
>>> torch.mm(a,b)
tensor([[1.1919, 1.1919],
[1.0837, 1.0837]])
>>> torch.matmul(a,b)
tensor([[1.1919, 1.1919],
[1.0837, 1.0837]])
>>> a@b
tensor([[1.1919, 1.1919],
[1.0837, 1.0837]])
dim>2时:
# Situation 1:前面维度一致
>>> a = torch.rand(4,3,28,64)
>>> b = torch.rand(4,3,64,32)
>>> torch.matmul(a,b).shape
torch.Size([4, 3, 28, 32])
# Situation 2:前面维度不一致,但是符合Broadcasting机制,会自动做广播,然后相乘
>>> a = torch.rand(4,3,28,64)
>>> b = torch.rand(4,1,64,32)
>>> torch.matmul(a,b).shape
torch.Size([4, 3, 28, 32])
更多内容可以前往官网查看:
https://pytorch.org/
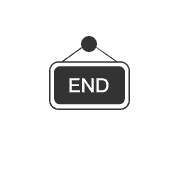
本篇文章来源于微信公众号: 码农设计师