本系列文章配套代码获取有以下两种途径:
-
通过百度网盘获取:
链接:https://pan.baidu.com/s/1XuxKa9_G00NznvSK0cr5qw?pwd=mnsj
提取码:mnsj
-
前往GitHub获取:
https://github.com/returu/PyTorch
Tensor的数据类型:
-
torch.float32 / torch.float: 32位浮点数,张量的默认数据类型。这是深度学习中最常用的数据类型,因为它提供了足够的精度来表示大多数网络参数和激活值。 -
torch.float64 / torch.double: 64位双精度浮点数。这种类型提供了更高的精度,但也需要更多的存储空间,它通常用于需要更高数值稳定性的科学计算。 -
torch.float16 / torch.half: 16位半精度浮点数。这种类型在标准CPU中并不存在,而是由现代GPU提供的。该类型在需要减少内存使用或加快计算速度时很有用,但可能会有精度损失。 -
torch.int8: 8位有符号整数。 -
torch.uint8: 8位无符号整数(0到255)。常用于图像处理中表示像素值。 -
torch.int16 / torch.short: 16位有符号整数。整数张量常用于索引或表示不能是浮点数的数据。 -
torch.int32 / torch.int: 32位有符号整数。 -
torch.int64 / torch.long: 64位有符号整数。当整数范围超过32位时,可以使用此类型。 -
torch.bool: 布尔类型,用于存储True或False值。
完整的数据类型介绍可以查看官方文档:
https://pytorch.org/docs/stable/tensors.html#data-types
>>> tensor = torch.rand(2,3)
>>> tensor
tensor([[0.4501, 0.0196, 0.0087],
[0.6253, 0.1520, 0.5375]])
>>> tensor.dtype
torch.float32
# 将默认数据类型设置为float64
>>> torch.set_default_tensor_type('torch.DoubleTensor')
>>> tensor_new = torch.rand(2,3)
>>> tensor_new
tensor([[0.4922, 0.3190, 0.8848],
[0.4611, 0.0300, 0.3920]])
>>> tensor_new.dtype
torch.float64
指定和更改Tensor的数据类型:
-
创建时指定数据类型:
# 创建一个float32类型的Tensor
>>> float_tensor = torch.tensor([1.0, 2.0, 3.0], dtype=torch.float32)
>>> float_tensor.dtype
torch.float32
# 创建一个int64类型的Tensor
>>> int64_tensor = torch.tensor([1, 2, 3], dtype=torch.int64)
>>> int64_tensor.dtype
torch.int64
也可以通过type()方法获取,或者使用isinstance()方法进行数据类型检验。
# 使用.type()方法可以返回一个str
>>> float_tensor.type()
'torch.FloatTensor'
# 使用isinstance()方法进行数据类型检验
>>> isinstance(int64_tensor , torch.LongTensor)
True
-
更改现有Tensor的数据类型:
# 将float32转换为float64类型
>>> double_tensor = float_tensor.double()
>>> double_tensor.dtype
torch.float64
# 将int64转换为int16类型
>>> int16_tensor = int64_tensor.short()
>>> int16_tensor.dtype
torch.int16
# 将float32转换为float16类型
>>> half_tensor = float_tensor.to(torch.half)
>>> half_tensor.dtype
torch.float16
# 将int64转换为int32类型
>>> int32_tensor = int64_tensor.type(torch.int)
>>> int32_tensor.dtype
torch.int32
>>> tensor_1 = torch.rand(5 , dtype=torch.float64)
>>> tensor_1
tensor([0.6739, 0.8314, 0.5675, 0.2497, 0.8152])
>>> tensor_2 = tensor_1.to(dtype=torch.int16)
>>> tensor_2
tensor([0, 0, 0, 0, 0], dtype=torch.int16)
>>> tensor_3 = tensor_1 + tensor_2
>>> tensor_3
tensor([0.6739, 0.8314, 0.5675, 0.2497, 0.8152])
>>> tensor_3.dtype
torch.float64
更多内容可以前往官网查看:
https://pytorch.org/
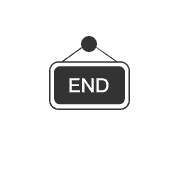
本篇文章来源于微信公众号: 码农设计师