Python受欢迎的原因之一就是其计算生态丰富,据不完全统计,Python 目前为止有约13万+的第三方库。
本系列将会陆续整理分享一些有趣、有用的第三方库。
-
通过百度网盘获取:
链接:https://pan.baidu.com/s/1FSGLd7aI_UQlCQuovVHc_Q?pwd=mnsj
提取码:mnsj
-
前往GitHub获取:
https://github.com/returu/Python_Ecosystem
pip install gTTS
https://github.com/pndurette/gTTS
gTTS提供了命令行工具和Python库两种使用方式。
-
命令行使用:
gtts-cli [OPTIONS] <text>
-
-f, –file <file>:从<file>读取,而不是<text>。 -
-o, –output <file>:写入<file>,而不是标准输出。 -
-s, –slow:读取速度更慢。 -
-l, –lang <lang>:IETF语言标签。指定语音语言。使用–all列出所有已记录的标签。默认值为‘en’。 -
–t, –tld <tld>:Google主机的顶级域名(例如https://translate.google.<tld>)。默认值为‘com’。 -
–nocheck:禁用严格的IETF语言标签检查,允许使用未记录的标签。 -
–all:打印所有已记录的IETF语言标签并退出。 -
–debug:显示调试信息。 -
–version:显示版本并退出。
# 列出可用语言:
gtts-cli --all
# 将“hello”读取到hello.mp3:
gtts-cli 'hello' --output hello.mp3
# 将“你好”读取到你好.mp3(使用普通话,通过google.com.hk):
gtts-cli '你好' --tld .com.hk --lang zh-CN --output 你好.mp3
# 将“slow”以较慢的速度读取到slow.mp3:
gtts-cli 'slow' --slow --output slow.mp3
# 将“hello”读取到标准输出:
gtts-cli 'hello'
gtts-cli 'hello' | play -t mp3 -
-
Python模块使用:
from gtts import gTTS
import os
# 输入文本
text = "Hello World!"
# 创建gTTS对象(India区域口音)
tts = gTTS(text=text, lang='en', slow=True, tld='co.in')
# 保存为MP3文件
tts.save("output.mp3")
# 在Windows上播放
os.system("start output.mp3")
# 在Mac上播放
# os.system("afplay output.mp3")
# 在Linux上播放
# os.system("mpg321 output.mp3")
-
text:要转换的文本内容(字符串)。需要注意的是,Google的API可能对单次请求的文本长度有限制,过长的文本需要分多次处理。 -
lang:语言代码。gTTS支持多种语言,例如英语(‘en’)(默认)、中文简体(‘zh-cn’)、中文繁体(‘zh-tw’)、日语(‘ja’)、法语(‘fr’)、西班牙语(‘es’)等。完整列表可通过Google Translate的API文档查询。 -
slow:发音速度(旧版本支持)。False为正常速度,True为慢速(某些语言可能不支持)。 -
tld:域名后缀(可选)。例如:‘com’、‘co.uk’,用于调整发音的区域口音。
gtts.tokenizer
模块是gTTS
(Google Text-to-Speech)库的核心组件,用于对文本进行预处理和分词。它提供了默认的预处理和分词功能,并允许用户自定义这些功能,以满足不同语言和文本格式的需求。
-
预处理(Pre-processing):
-
tone_marks:在语气修饰标点符号后添加空格,以便分词器正确处理。 -
end_of_line:重新组合被换行符截断的单词,移除行尾的连字符。 -
abbreviations:移除某些已知缩写后的句号,避免分词器错误处理。 -
word_sub:进行逐词替换,纠正发音或替换特定词汇。
-
gtts.tokenizer.core.PreProcessorRegex类(用于基于正则表达式的替换,类似于re.sub的用法); -
gtts.tokenizer.core.PreProcessorSub类(用于逐词替换)。
from gtts.tokenizer import pre_processors
import gtts.tokenizer.symbols
# 添加自定义替换规则
gtts.tokenizer.symbols.SUB_PAIRS.extend([
('sub.', 'submarine'), # 替换 "sub." 为 "submarine"
('Mr.', 'Mister') # 替换 "Mr." 为 "Mister"
])
# 测试文本
text = "Mr. Smith works on a sub. project."
processed_text = pre_processors.word_sub(text)
print(processed_text)
# 输出:"Mister Smith works on a submarine project."
-
分词(Tokenizing):
-
tone_marks:处理语气修饰标点符号。 -
period_comma:处理句号和逗号,避免在缩写或数字中错误切割。 -
other_punctuation:处理其他标点符号,自然地插入语音停顿。 -
colon:处理冒号,避免在时间表示中切割。
-
在最接近但位于第100个字符之前的最后一个空格字符处分割; -
如果没有空格,则在第100个和第101个字符之间分割。
示例代码:
自定义分词器,处理特定的标点符号。
from gtts.tokenizer import Tokenizer, pre_processors, tokenizer_cases
from gtts import gTTS
# 创建自定义分词器
custom_tokenizer = Tokenizer(
regex_funcs=[
tokenizer_cases.period_comma, # 句号和逗号
tokenizer_cases.colon, # 冒号
tokenizer_cases.tone_marks, # 问号和感叹号
tokenizer_cases.other_punctuation # 其他标点符号
]
)
# 原始文本
text = "Hello, this is an example. It includes abbreviations like Dr. and Mr.. "
"Are you ready? Let's go! This is an important announcement: "
"Please pay attention."
# 使用默认分词器
tts = gTTS(text)
tts.save("example.mp3")
# 使用自定义分词器
tts = gTTS(text, tokenizer_func=custom_tokenizer.run) # 将自定义分词器传递给 gTTS
tts.save("custom_tokenizer_example.mp3")
# 验证分词效果
processed_text = custom_tokenizer.run(text)
print("原始文本:", text)
print("处理后的文本:", processed_text)
# 原始文本: Hello, this is an example. It includes abbreviations like Dr. and Mr.. Are you ready? Let's go! This is an important announcement: Please pay attention.
# 处理后的文本: ['Hello', 'this is an example', 'It includes abbreviations like Dr', 'and Mr.', 'Are you ready?', "Let's go!", 'This is an important announcement', ' Please pay attention.']
更多内容可以前往官方文档查看:
https://gtts.readthedocs.io/en/latest/
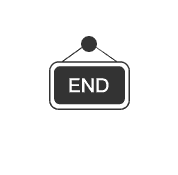

本篇文章来源于微信公众号: 码农设计师